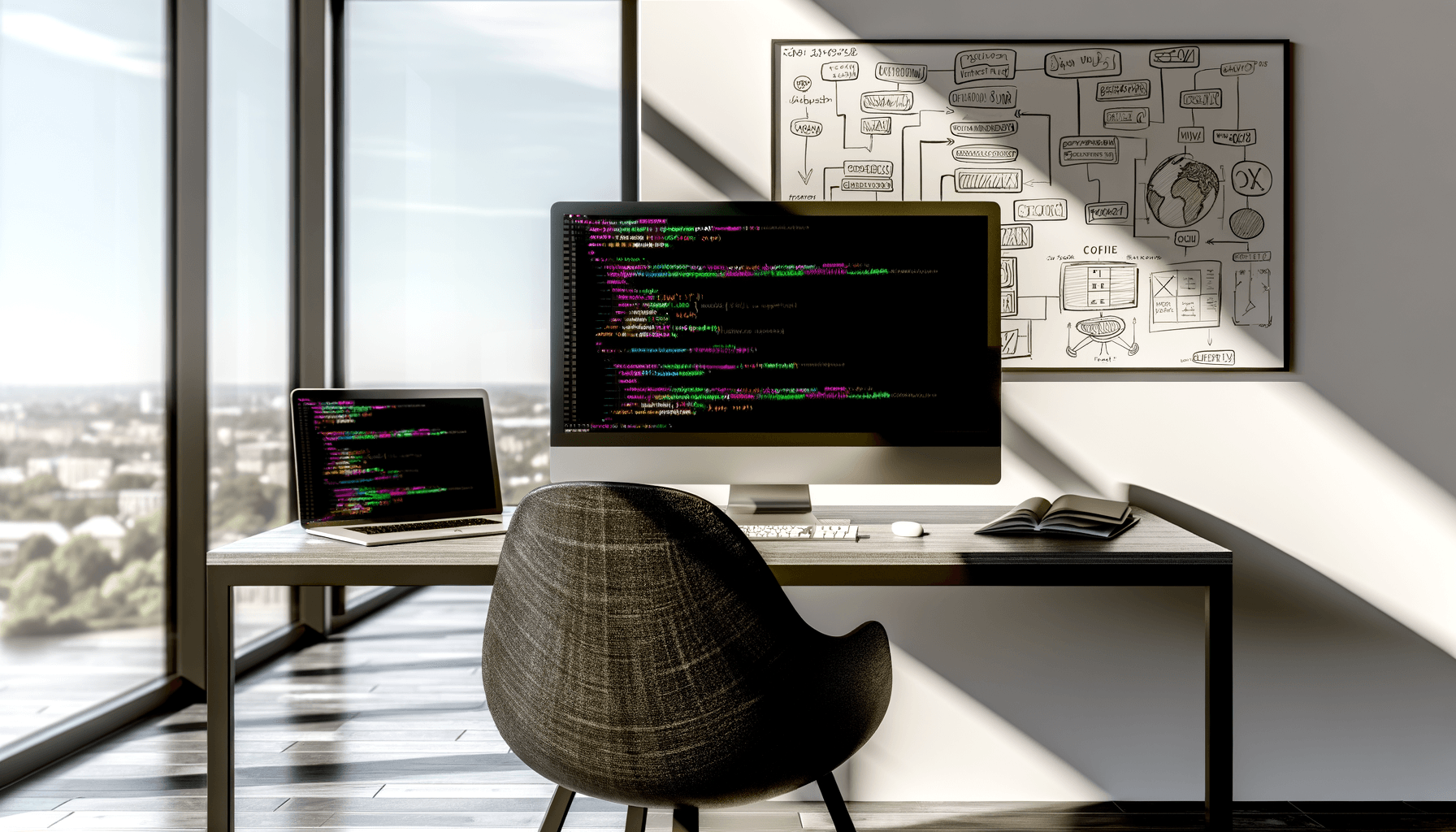
In the rapidly evolving world of web development, choosing the right technology stack is crucial for building scalable, efficient, and high-performance applications. Among the myriad of options available, Node.js has emerged as a preferred choice for developers and businesses alike. In this ultimate guide to Node JS web development services, we will explore why Node.js has gained such popularity, delve into its features and benefits, and provide insights into how you can leverage it for your web development projects.
Introduction to Node JS Web Development Services
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a browser. Developed by Ryan Dahl in 2009, Node.js extends the capabilities of JavaScript from being a client-side scripting language to a robust server-side programming language. This transformation has opened up new horizons for developers, allowing them to build full-stack applications using a single language.
Node JS web development services encompass a wide range of solutions aimed at creating server-side applications, APIs, and network tools. The use of Node.js in web development offers numerous advantages, such as improved performance, scalability, and the ability to handle real-time data. This guide aims to provide a comprehensive understanding of Node.js and how it can be utilized effectively in web development.
Why Choose Node JS for Web Development?
1. Single Language for Full-Stack Development
One of the most significant advantages of Node JS web development services is the ability to use JavaScript for both client-side and server-side programming. This unification simplifies the development process, as developers can work with a single language across the entire stack. This not only reduces the learning curve but also streamlines communication between front-end and back-end teams.
2. High Performance
Node.js is built on the V8 JavaScript engine developed by Google, which compiles JavaScript into native machine code. This results in exceptional performance and speed. Node.js operates on a non-blocking, event-driven architecture, enabling it to handle multiple connections simultaneously without incurring high overheads. This makes Node.js particularly suitable for building high-performance applications that require low latency and responsiveness.
3. Scalability
Scalability is a critical factor for modern web applications, and Node JS web development services excel in this regard. Node.js applications are designed to be scalable by default, thanks to their event-driven nature and the ability to utilize asynchronous programming. The use of microservices architecture further enhances the scalability of Node.js applications, allowing them to handle increasing loads efficiently.
4. Rich Ecosystem
Node.js boasts a vibrant ecosystem with a vast collection of libraries and modules available through npm (Node Package Manager). This extensive library of open-source packages accelerates development by providing pre-built solutions for common tasks, reducing the need for reinventing the wheel. This ecosystem enables developers to quickly integrate third-party services, APIs, and tools, thus enhancing the functionality of their applications.
5. Active Community
Node.js has a robust and active community that continuously contributes to its growth and improvement. The community-driven nature of Node.js ensures that it remains up-to-date with the latest trends and technologies. Developers have access to numerous resources, tutorials, and forums where they can seek help, share knowledge, and collaborate with others.
Core Features of Node JS Web Development Services
1. Event-Driven Architecture
Node.js follows an event-driven, non-blocking architecture that allows it to handle multiple requests simultaneously without waiting for a task to complete before moving on to the next one. This makes it ideal for building applications that require real-time data processing, such as chat applications, online gaming, and collaborative tools.
2. Asynchronous I/O
Node.js uses asynchronous input/output operations, which means tasks can be executed independently without blocking the execution of other operations. This results in improved performance and efficiency, as applications can handle multiple requests concurrently without being held up by slow operations.
3. Microservices Support
Node JS web development services often employ a microservices architecture, where applications are broken down into smaller, independent services that communicate with each other. This architecture enhances scalability, maintainability, and flexibility, enabling developers to build complex applications that can be easily scaled and managed.
4. Cross-Platform Compatibility
Node.js is compatible with various operating systems, including Windows, macOS, and Linux. This cross-platform compatibility ensures that Node.js applications can be deployed and run on a wide range of devices and environments, making it a versatile choice for web development.
5. Real-Time Data Handling
Node.js is renowned for its ability to handle real-time data efficiently. It is commonly used in applications that require constant data updates, such as live streaming, online collaboration tools, and IoT devices. The non-blocking architecture ensures that data can be processed and delivered in real-time, providing a seamless user experience.
Building a Node JS Web Application: A Step-by-Step Guide
Step 1: Setting Up the Development Environment
To begin developing with Node.js, you need to set up your development environment. This involves installing Node.js and npm on your machine. You can download the latest version of Node.js from the official website and follow the installation instructions for your operating system.
Step 2: Initializing a New Node.js Project
Once Node.js is installed, you can create a new project by initializing a package.json file. This file contains essential information about your project, such as its name, version, dependencies, and scripts. You can generate a package.json file by running the following command in your project directory:
npm init
Follow the prompts to provide the necessary details for your project.
Step 3: Installing Essential Packages
Node JS web development services rely on various packages and modules to enhance functionality. Some of the essential packages for a Node.js web application include Express.js for building web servers, Mongoose for database integration, and Socket.io for real-time communication. You can install these packages using npm:
npm install express mongoose socket.io
Step 4: Building the Server
With the necessary packages installed, you can start building the server for your application. Use Express.js to create a server and define routes to handle incoming requests. Here’s a basic example of setting up a Node.js server using Express:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Step 5: Implementing Database Integration
Most web applications require a database to store and retrieve data. Node.js supports various databases, including MongoDB, PostgreSQL, and MySQL. Mongoose is a popular ODM (Object Data Modeling) library for MongoDB that simplifies database operations. You can integrate MongoDB into your Node.js application using Mongoose:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/mydatabase', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
const db = mongoose.connection;
db.on('error', console.error.bind(console, 'connection error:'));
db.once('open', () => {
console.log('Database connected');
});
Step 6: Adding Real-Time Features
To incorporate real-time features into your application, you can use Socket.io. This library enables bidirectional communication between the server and clients, making it ideal for real-time applications. Here’s a simple example of using Socket.io with Node.js:
const http = require('http').createServer(app);
const io = require('socket.io')(http);
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('message', (msg) => {
io.emit('message', msg);
});
});
http.listen(port, () => {
console.log(`Server is running with Socket.io on http://localhost:${port}`);
});
Step 7: Testing and Deployment
Before deploying your Node.js application, it’s essential to conduct thorough testing to ensure it functions as expected. You can use testing frameworks like Mocha and Chai to automate testing processes. Once testing is complete, you can deploy your application to a server or cloud platform, such as AWS, Heroku, or DigitalOcean.
Best Practices for Node JS Web Development Services
1. Code Organization and Structure
Organizing your codebase effectively is crucial for maintainability and scalability. Follow a modular approach by breaking down your application into smaller, independent modules. Use a consistent folder structure and naming conventions to make your code easier to navigate.
2. Error Handling
Implement robust error handling mechanisms to ensure your application can gracefully handle unexpected errors and exceptions. Use try-catch blocks, middleware, and logging tools to capture and manage errors effectively.
3. Security Best Practices
Security should be a top priority in web development. Follow security best practices, such as validating user input, implementing authentication and authorization, and using HTTPS to encrypt data transmission. Stay updated with security patches and updates for Node.js and its dependencies.
4. Performance Optimization
Optimize the performance of your Node.js application by minimizing the use of synchronous operations, reducing the number of API calls, and using caching mechanisms. Monitor application performance using tools like New Relic or AppDynamics to identify and address bottlenecks.
5. Continuous Integration and Deployment
Implement continuous integration and deployment (CI/CD) pipelines to automate the testing, building, and deployment processes. This ensures that your application is consistently delivered with high quality and reduces the risk of manual errors.
Conclusion
Node JS web development services offer a powerful and versatile solution for building modern web applications. With its event-driven architecture, high performance, and vast ecosystem, Node.js empowers developers to create scalable, efficient, and real-time applications. By following best practices and leveraging the rich ecosystem of libraries and tools, businesses can harness the full potential of Node.js to deliver exceptional web experiences.
Whether you’re a startup looking to build a new application or an established company aiming to enhance existing solutions, Node JS web development services provide the flexibility and scalability needed to succeed in today’s digital landscape. By embracing Node.js, you can stay ahead of the competition and deliver innovative solutions that meet the demands of modern users.